Higher Order Functions
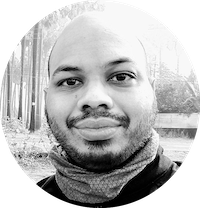
Higher Order Function is a Functional Programming concept which is very helful in terms of reusability & readability.
TL;DR
Higher Order Functions are simply functions that take other functions as arguments or return a function as result. A quote from Wikipedia
A higher-order function is a function that does at least one of the following:
- takes one or more functions as arguments (i.e. procedural parameters),
- returns a function as its result.
Our piece
Now what it means is that, the Higher Order Function is going to provide us some additional functionality around our own function that we pass to it.
Wrapper is how I make out it & remember.
Wrapper: One who, or that which, wraps.
The most common example we see with JavaScript is the Array map
function.
It takes a function that is run on each element of the array to return a new array. Let’s break it down:
- It iterates on each element of array
- It executes the function passed on argument on each element
- It returns a new array
Some actuals
Consider an example, we have an array of numbers & we want to find square of each of them.
// create array of numbers
const numbers = [ 2, 3, 4, 5 ];
// Function that takes a number &
// returns result that is the product of number with itself (square)
const square = (num) => num * num;
// new array created using map function,
// that uses square function on each element in numbers array
const squared_numbers = numbers.map(aNumber => square(aNumber));
// printing the input & output arrays
console.log('Numbers: ', numbers);
console.log('Squared Numbers: ', squared_numbers);
Here is the output when we run it in browser console:
Some few more examples of Higher Order Functions too that we use daily but fail to notice them:
- filter
- reduce
- some
Similar concept is leveraged by React for Higher Order Components which I will cover in another article.